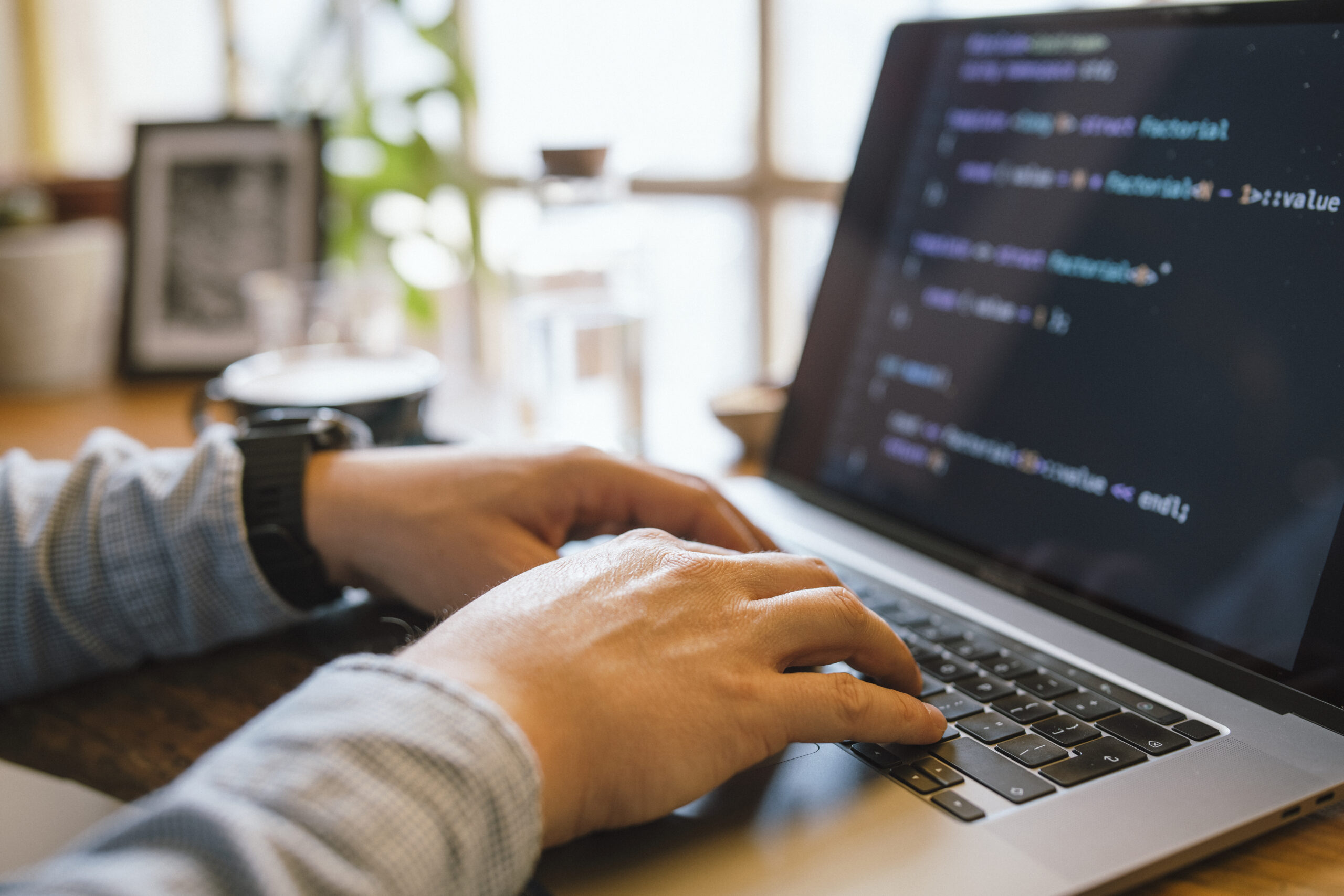
Debugging is Probably the most necessary — yet frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Finding out to Assume methodically to unravel challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your productiveness. Allow me to share many approaches to help you developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods developers can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is one Element of progress, being aware of how you can connect with it proficiently for the duration of execution is equally vital. Present day advancement environments come Outfitted with potent debugging abilities — but a lot of developers only scratch the floor of what these resources can perform.
Get, for example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools allow you to set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and perhaps modify code on the fly. When employed properly, they Permit you to notice precisely how your code behaves during execution, which happens to be a must have for tracking down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for front-finish developers. They assist you to inspect the DOM, check community requests, look at genuine-time effectiveness metrics, and debug JavaScript inside the browser. Mastering the console, sources, and network tabs can convert aggravating UI challenges into manageable responsibilities.
For backend or method-stage developers, resources like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Mastering these tools might have a steeper Discovering curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation Manage techniques like Git to be aware of code record, find the exact moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications usually means likely beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting making sure that when difficulties crop up, you’re not missing at the hours of darkness. The greater you already know your applications, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the situation. Ahead of jumping into the code or earning guesses, builders want to create a consistent ecosystem or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a match of likelihood, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected ample info, endeavor to recreate the issue in your neighborhood atmosphere. This might mean inputting the exact same information, simulating very similar user interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the edge circumstances or point out transitions involved. These exams not simply help expose the challenge but will also stop regressions Sooner or later.
Sometimes, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than specific configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. That has a reproducible state of affairs, You may use your debugging tools more successfully, check prospective fixes securely, and talk much more Obviously together with your group or customers. It turns an abstract criticism right into a concrete obstacle — Which’s the place developers thrive.
Read and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the concept very carefully and in whole. Several builders, particularly when below time tension, look at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the true root bring about. Don’t just copy and paste mistake messages into serps — study and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate triggered it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, and in Individuals conditions, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and up to date modifications while in the codebase.
Don’t ignore compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is Probably the most effective resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic details in the course of improvement, INFO for general situations (like prosperous start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your process. Target important events, condition modifications, enter/output values, and demanding decision factors inside your code.
Structure your log messages Obviously and consistently. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are achieved, and what branches of logic are executed—all devoid of halting the program. They’re Particularly important in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a very well-believed-out logging technique, you can decrease the time it will require to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To successfully establish and take care of bugs, developers should technique the procedure similar to a detective fixing a secret. This mindset assists break down intricate difficulties into workable pieces and follow clues logically to uncover the root result in.
Start out by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent data as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What could be producing this actions? Have any improvements not long ago been manufactured for the codebase? Has this concern occurred right before less than related conditions? The objective is to slender down options and discover possible culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled ecosystem. In case you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcome direct you nearer to the reality.
Fork out near attention to smaller specifics. Bugs frequently disguise while in the least anticipated places—just like a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and affected person, resisting the urge to patch The difficulty without having absolutely comprehension it. Temporary fixes may possibly disguise the true trouble, only for it to resurface later on.
Last of all, hold notes on Anything you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for long term troubles and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated devices.
Write Tests
Composing assessments is among the simplest methods to boost your debugging techniques and In general improvement effectiveness. Assessments not simply assistance catch bugs early but additionally serve as a safety Internet that provides you self confidence when building variations to your codebase. A nicely-tested application is easier to debug since it permits you to pinpoint just wherever and when a challenge takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated assessments can speedily reveal no matter whether a certain piece of logic is Operating as expected. When a test fails, you immediately know where by to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear soon after Formerly being preset.
Upcoming, integrate integration tests and close-to-conclusion assessments into your workflow. These assist ensure that many portions of your application work jointly easily. They’re especially practical for catching bugs that arise in complicated systems with many elements or products and services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and underneath what situations.
Writing assessments also forces you to definitely Consider critically about your code. To test a feature adequately, you will need to be familiar with its inputs, anticipated outputs, and edge cases. This amount of comprehension naturally sales opportunities to better code construction and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, writing tests turns debugging from a discouraging guessing game into a structured and predictable approach—serving to you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could commence overlooking clear problems or misreading code which you wrote just hours earlier. Within this state, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Strength along with a clearer mentality. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to maneuver all around, extend, or do a thing more info unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can educate you a thing important if you take some time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but those who continually master from their blunders.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It tends to make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.